Hey fellas, we’re back again and this time I want to kick things off by talking about a challenge I faced while building Django Templates: I had already built a basic framework (more on that later) when I wanted to copy the code of a free template to drastically improve the UI of my application. I found a decent template and copied the code … and to my surprise, absolutely nothing happened.
I spent a considerable amount of time over the last 3 days trying hopelessly to ascertain the root cause. It grew increasingly frustrating, and I was honestly at a loss. I went and checked all my settings, files, and URLs;Everything seemed perfectly fine, and yet my website looked like this :
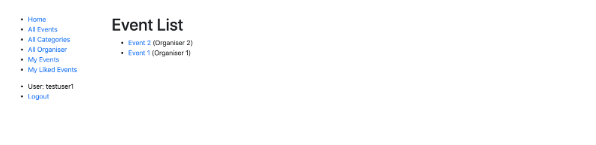
Instead of this:
The difference is obviously striking and I found the “bug” this morning — a stray quotation mark that caused all the code to be essentially a string. This is besides my earlier experiences with the templates, but it’s a frequent frustration too many of us know all too well, yet often overlook.
So now onto the main topic of this post, the usage of django templates.
I’ll only be talking about Django Template Language and Engine as the other alternatives lie outside the scope of what I’ve talked about so far. To understand what templates in Django are, we first need to understand why we need them in the first place.
“Being a web framework, Django needs a convenient way to generate HTML dynamically. The most common approach relies on templates. A template contains the static parts of the desired HTML output as well as some special syntax describing how dynamic content will be inserted. “ (pulled from this insightful piece)
I believe that’s a fairly clear and concise explanation of templates.
So, we essentially use templates for the creation of multiple pages that only differ by a slight amount of information.For example, if I wanted to create a template for listing an event, the simplest template I would create would be as follows:
{% extends “base_generic.html” %} {% block content %} <h1>Title: {{ event.name }}</h1> <p><strong>Organiser:</strong> <a href=”{% url ‘organiser-detail’ event.organiser.pk %}”>{{ event.organiser }}</a></p> <p><strong>Picture:</strong> <br><img src= {{ event.picture.url }} style=”width:400px;height:300px” > </p> <p><strong>Date and Time:</strong> {{ event.date}} {{event.time}}</p> <p><strong>Capacity:</strong> {{ event.capacity }}</p> <p><strong>Venue:</strong> {{ event.venue }}</p> <p><strong>Category:</strong> {% for category in event.category.all %} {{ category }}{% if not forloop.last %}, {% endif %}{% endfor %}</p> {% endblock %}
Whenever you create a new template always remember to do the following:
- That you actually have a view linked to the template. This may seem trivial,but we often forget to create the view if it is something simple and generic. In this case it is something as simple as:
class EventDetailView(generic.DetailView):model = Event
2. That you have added the template to the urls.py file:
urlpatterns += [path(‘event/<int:pk>’, views.EventDetailView.as_view(), name=’event-detail’),]
And that’s it. With that small amount of code, we can now generate event pages endlessly with the specific dynamic content. It’d look something similar to this:
So there you have it — Have fun creating templates and taking inspiration from already established HTML templates. Just a word of caution — Remember to configure your static files correctly and load the static information each time, and you’ll be creating web apps much better than me in absolutely no time.
Well see you again next week!
References:
https://docs.djangoproject.com/en/2.2/topics/templates/
https://docs.djangoproject.com/en/2.2/intro/tutorial03/